Remix Run Resource Routes
One of the great things about Remix Run is the ability to create resource routes. A resource route is a route is that is not part of your applications UI but instead is available as an API endpoint. This can be a great way to automate repetitive tasks.
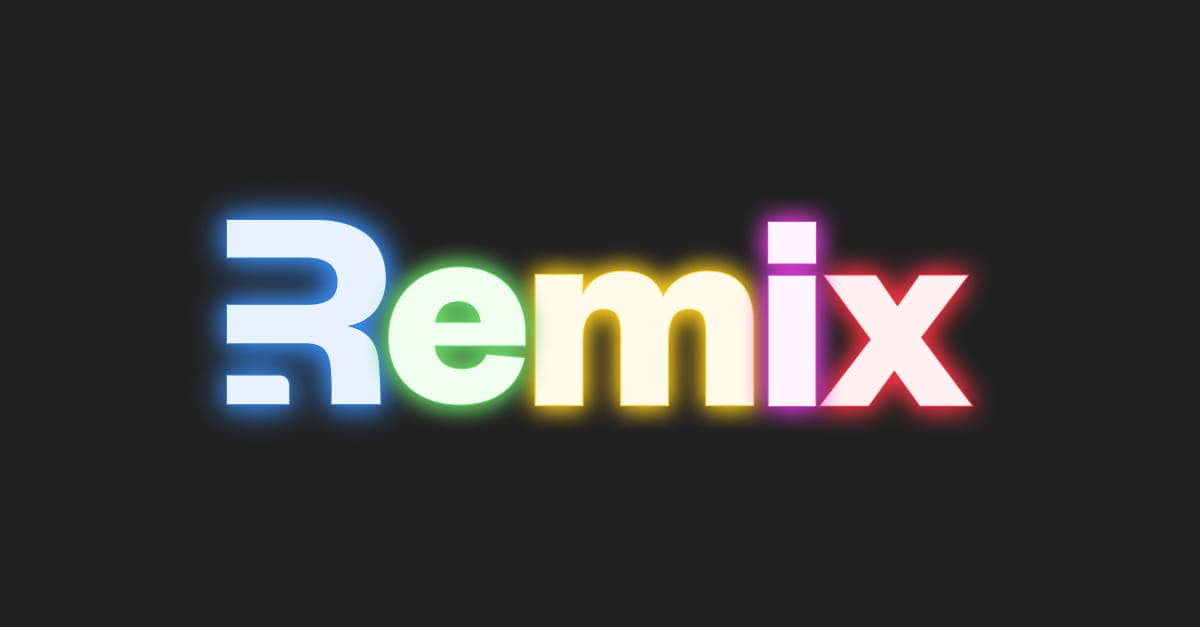
Remix Run - The Framework
Remix Run is a framework for building web applications. It is built on top of React and React Router. It is a full stack framework that includes a server side rendering engine, a client side rendering engine, and a routing engine. It also includes a build tool that can be used to build your application for production.
Aside from the framework itself, Remix Run also includes a CLI tool that can be used to create new projects, run the development server, and build your application for production.
Remix Run - A Resource Route
In what cases would a Resource Route be beneficial? Well, that would be any kind of task that you would want the server to do without it being part of the UI. For example, if you wanted to create a pdf from a database query, you could create an resource route for that.
Remix Run - Creating a Resource Route
Creating a resource route is very simple. All you need to do is create a file in the routes
directory and export a function called loader
. This function will be called when the route is requested. The function should return an object with a status
and body
property. The status
property should be the HTTP status code that you want to return and the body
property should be the body of the response.
// routes/pdf.ts
import {type LoaderArgs} from '@remix-run/node'
export async function loader({request}: LoaderArgs) {
return {
status: 200,
body: 'Hello World',
}
}
Remix Run - Using a Resource Route
Using a resource route is just as simple as creating one. All you need to do is make a request to the route. For example, if you created a resource route called pdf
you could make a request to it like this:
const pdfRes = await fetch('/pdf')
const pdf = await pdfRes.json() // Hello World
Variations
There are a few variations of resource routes. Remix has two kinds of server functions that listen at the same path. The first is a loader function. It accepts all GET
requests and returns a response. The second is an action function that accepts all POST
, OPTIONS
, DELETE
, and PUT
requests and returns a response.
// routes/pdf.ts
import {type ActionArgs} from '@remix-run/node'
import {generateReport} from '../utils/pdf'
export async function action({request}: ActionArgs) {
// can handle POST, OPTIONS, DELETE, and PUT requests
// and do different things based on the request method
if(request.method === 'POST') {
const formData = await request.formData()
const name = formData.get('name')
const report = await generateReport(name)
return new Response(report, {
headers: {
'Content-Type': 'application/pdf',
},
})
} else if(request.method === 'OPTIONS') {
return new Response(null, {
headers: {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Methods': 'POST, OPTIONS',
'Access-Control-Allow-Headers': 'Content-Type',
},
})
} // etc...
}
Remix Run - Conclusion
Remix Run is a great framework for building web applications. It is built on top of React and React Router. It is a full stack framework that includes a server side rendering engine, a client side rendering engine, and a routing engine. It also includes a build tool that can be used to build your application for production.